Testing is a critical part of ensuring your web application works as expected. Whether you’re building a complex SaaS platform or a small blog, writing good tests helps catch bugs early and makes your code more maintainable.
For frontend testing in a Next.js project, Jest and React Testing Library are powerful tools that allow you to test your components, user interactions, and API integration effectively. Let’s explore how you can set up a robust testing environment and write meaningful tests for your frontend components.
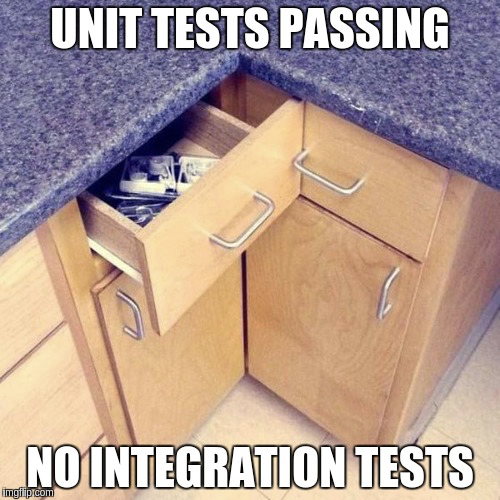
Setting Up Frontend Testing for Next.js
To start testing your Next.js project, you need to set up the right tools and configurations. Here’s how:
1. Install Dependencies
First, install the necessary libraries:
npm install --save-dev jest @testing-library/react @testing-library/jest-dom @testing-library/user-event msw
2. Configure Jest
Next, set up Jest to work seamlessly with Next.js. Add a jest.config.js file:
const nextJest = require('next/jest');
const createJestConfig = nextJest({ dir: './' });
const customJestConfig = {
setupFilesAfterEnv: ['<rootDir>/jest.setup.js'],
testEnvironment: 'jest-environment-jsdom',
};
module.exports = createJestConfig(customJestConfig);
3. Setup Testing Library:
Add global configurations in jest.setup.js:
import '@testing-library/jest-dom';
import 'whatwg-fetch'; // If you're using fetch in your app
4. Mock Next.js Features:
If you’re using Next.js hooks like useRouter, you’ll want to mock them:
jest.mock('next/router', () => ({
useRouter: jest.fn().mockReturnValue({
push: jest.fn(),
}),
}));
Writing Tests for Frontend Components
Let’s walk through testing a simple component called ContactForm. The component allows users to fill in their name and email and submit the form.
The Component
Here’s a sample ContactForm component:
import React, { useState } from 'react';
const ContactForm = () => {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const [message, setMessage] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
alert(`Submitted: ${name}, ${email}`);
};
return (
<form onSubmit={handleSubmit}>
<input
data-testid="name-input"
type="text"
placeholder="Your Name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<input
data-testid="email-input"
type="email"
placeholder="Your Email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<button data-testid="submit-button" type="submit">
Submit
</button>
</form>
);
};
export default ContactForm;
Testing the Component
Now let’s write tests to validate the behavior of the ContactForm.
Testing Initial Rendering
A simple test to verify that the form renders correctly:
import { render, screen } from '@testing-library/react';
import ContactForm from '../components/ContactForm';
test('renders the form fields and button', () => {
render(<ContactForm />);
expect(screen.getByPlaceholderText('Your Name')).toBeInTheDocument();
expect(screen.getByPlaceholderText('Your Email')).toBeInTheDocument();
expect(screen.getByTestId('submit-button')).toBeInTheDocument();
});
Testing User Interactions
Next, simulate user interactions to test the form functionality:
import userEvent from '@testing-library/user-event';
test('allows users to type into form fields', async () => {
render(<ContactForm />);
const nameInput = screen.getByTestId('name-input');
const emailInput = screen.getByTestId('email-input');
await userEvent.type(nameInput, 'John Doe');
await userEvent.type(emailInput, '[email protected]');
expect(nameInput).toHaveValue('John Doe');
expect(emailInput).toHaveValue('[email protected]');
});
Mocking API Calls
If your component fetches data or submits forms to an API, you can use MSW (Mock Service Worker) to mock the API responses. This ensures your tests remain independent of external services.
import { setupServer } from 'msw/node';
import { rest } from 'msw';
const server = setupServer(
rest.post('/api/contact', (req, res, ctx) => {
return res(ctx.status(200), ctx.json({ success: true }));
})
);
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
test('submits form data to the API', async () => {
render(<ContactForm />);
await userEvent.type(screen.getByTestId('name-input'), 'John Doe');
await userEvent.type(screen.getByTestId('email-input'), '[email protected]');
await userEvent.click(screen.getByTestId('submit-button'));
// Assert that the mocked API endpoint was called
expect(server.events.requestStart).toBeCalledWith(
expect.objectContaining({ body: { name: 'John Doe', email: '[email protected]' } })
);
});
Tips I’ve Learned Along the Way
1. Keep Tests Small: Focus on one thing per test. It makes debugging easier when something fails.
2. Mock What You Can: Mock APIs, hooks, and anything else that might introduce unpredictability.
3. Write Tests for Important Stuff: Don’t try to test everything—start with critical features and expand as needed.
4. Use waitFor for Async Stuff: If something happens after a delay (like fetching data), wrap your assertions in waitFor.
import { waitFor } from '@testing-library/react';
test('displays data after loading', async () => {
render(<ContactForm />);
await waitFor(() => {
expect(screen.getByText('Your Name')).toBeInTheDocument();
});
});
Final Thoughts
Writing tests might feel tedious at first, but it’s worth it. It gives you confidence to deploy changes, refactor code, and sleep at night knowing your app won’t break unexpectedly.
If you’re new to testing, don’t worry. Start small, keep practicing, and before you know it, writing tests will feel like second nature.
Got questions or tips to share? Let me know! I’d love to hear how you approach testing in your projects. ?